byteauth-laravel
Description
ByteAuth-Laravel is a plug and play Laravel package that integrates Byte Federal's fast authentication system into your Laravel application, leveraging bitcoin cryptographic (ECDSA/SHA256) standards. This advanced package not only offers a seamless way to onboard users and enable secure sign-in functionalities, akin to the Webauthn standard, but it also introduces several groundbreaking features:
- It ensures that cryptographic keys are backed up for added security,
- Operates independently of the user's operating system and instead uses a smart-phone as universal access key,
- Incorporates a user identity check to thwart fraudulent users including a liveness check to verify the presence of real human beings during authentication.
- Additionally, it furnishes users with a wallet that supports lightning and bitcoin transactions, enhancing the overall user experience (LNUrl, micropayments, etc).
By integrating ByteAuth-Laravel, you can easily implement a state-of-the-art, comprehensive authentication system in your Laravel projects, harnessing the robustness and security of bitcoin's cryptographic infrastructure.
Features
- Fast Authentication: Utilize Byte Federal's rapid authentication process to enhance user experience.
- Bitcoin Cryptographic Standards: Benefit from the high security of bitcoin cryptographic practices.
- Easy Integration: Seamlessly integrate with Laravel applications, providing a Livewire component for both front-end and back-end operations.
- Sample Login Page: Get started quickly with a sample login landing page tailored for Laravel applications.
- Webhook Support: Includes a
WebhookController
for handling authentication callbacks.
Installation
Follow the instructions on Github to install and configure byteauth-laravel in your Laravel project.
After Installation
Once you have installed the package which might look something like this:
sudo composer require bytefederal/byteauth-laravel:@dev
you then proceed to add the main routes to your web.php routing file:
...
//ByteAuth
Route::post('/webhook/registration', [WebhookController::class, 'handleRegistration']);
Route::post('/webhook/login', [WebhookController::class, 'handleLogin']);
Route::get('/check', [WebhookController::class, 'check']);
Route::get('/byte', [WebhookController::class, 'sample']);
...
Notice that you will need to include the WebhookController
class at the top of your routing file like this:
...
use ByteFederal\ByteAuthLaravel\Controllers\WebhookController;
...
Make sure you have set up your webhook endpoint in ByteWallet. See here how to do that
With both registration and login endpoints registered make sure you export the byteauth configuration
php artisan vendor:publish --tag=byteauth-config
php artisan route:cache
php artisan config:cache
and enter your domain and api key that you created in your webwallet profile.
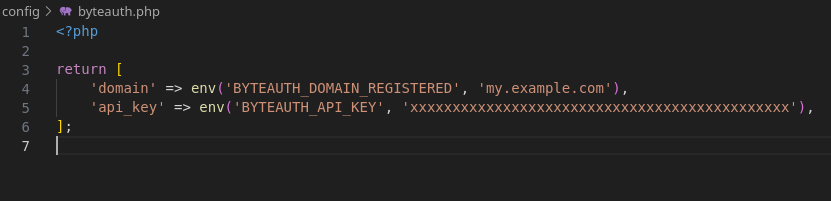
At this point, with the route and view's cache cleared, you should be able to simply head over to your project's url my.website.com/byte
and find a beautiful simple QR-code based login sample page that looks like this:
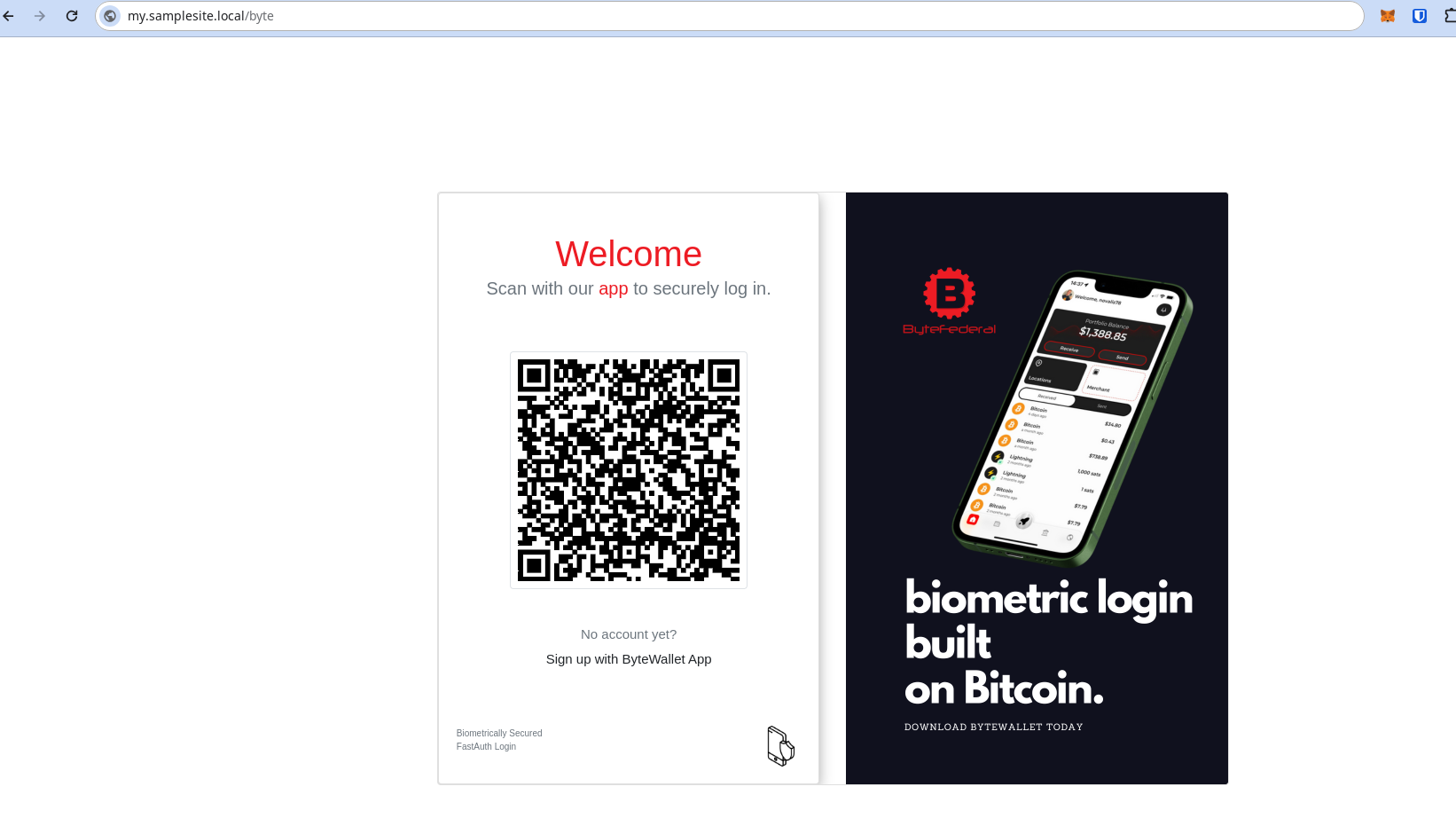
So what's going on here? Let's take a moment to understand what we did!
With the installation of the byteauth-laravel
composer plugin we now have two webhooks that will receive user information from anyone scanning our QR code with their ByteWallet. Our website receives the information and, in the case of a new user, hits our registration webhook. In case of an existing user, the login webhook.
A user who logs in, someone we have seen before, will get automagically authenticated against Laravel's internal authentication system (breeze) and gets forwarded to our specific dashboard etc.
A new user, never seen before, is someone we can intercept and navigate to a page where we ask for a little more identifying information - if we wish - and attach that information to a profile or user table. But we don't have to! In theory we have enough data to create a new user account (which is the default behavior) and let the user redirect to the our website's dashboard.
So what are the next steps?
Login Page Refactoring: we might want to integrate the QR code / Livewire option into our existing login page (see below for an example)
Advertise: we might want to tell users about this new and passwordless way to log in. Checkout badges and examples to add to your site which link to Bytewallet so your users can download ByteFederal's free crypto wallet.
Sample Integration
Let's take a sample login page that your website might already have, like the following:
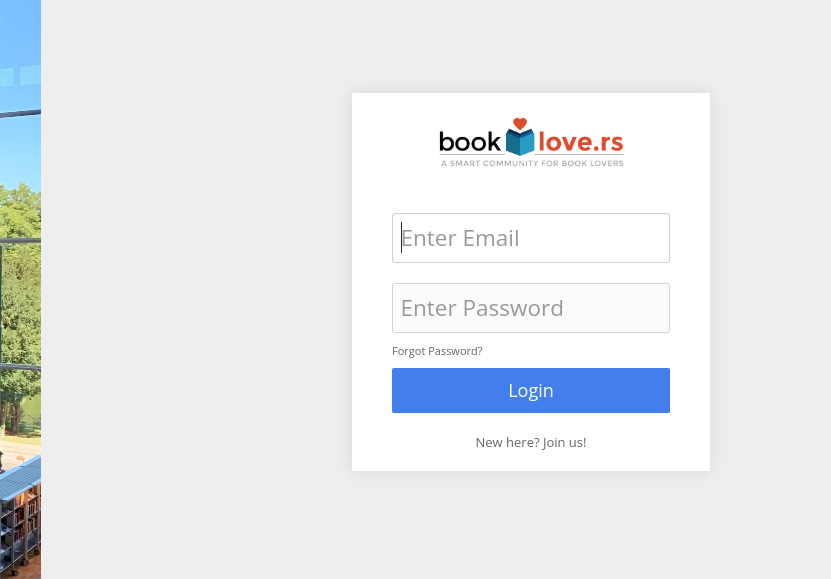
With just a few CSS tweaks, you could add the Livewire QR code generated plugin to the "back" of your login/registration field:
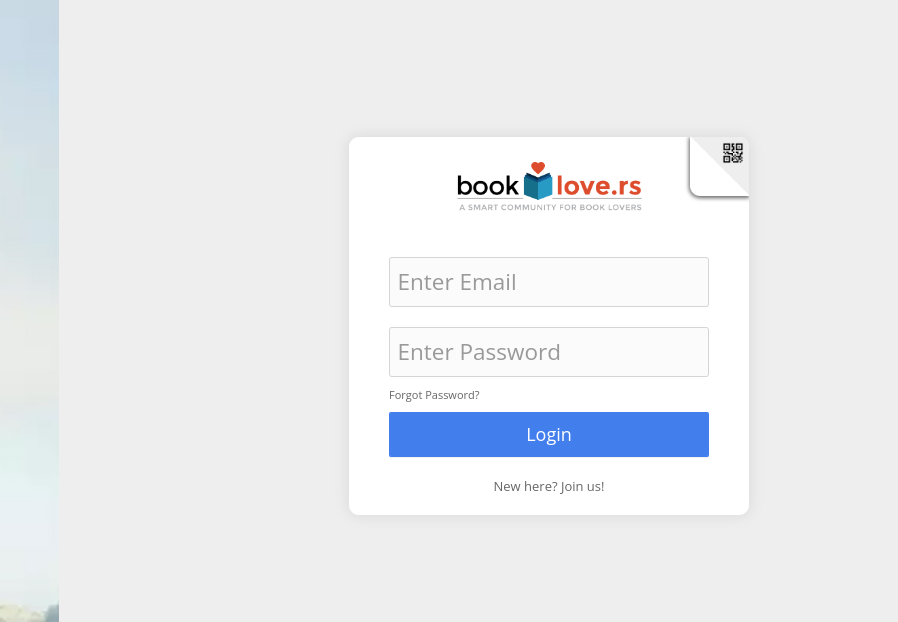
Now, when a customer visits your landing page, they'll be greeted with a regular email based login system. However, you are starting to introduce the more superior password-less biometric QR-code based login system in the "background":
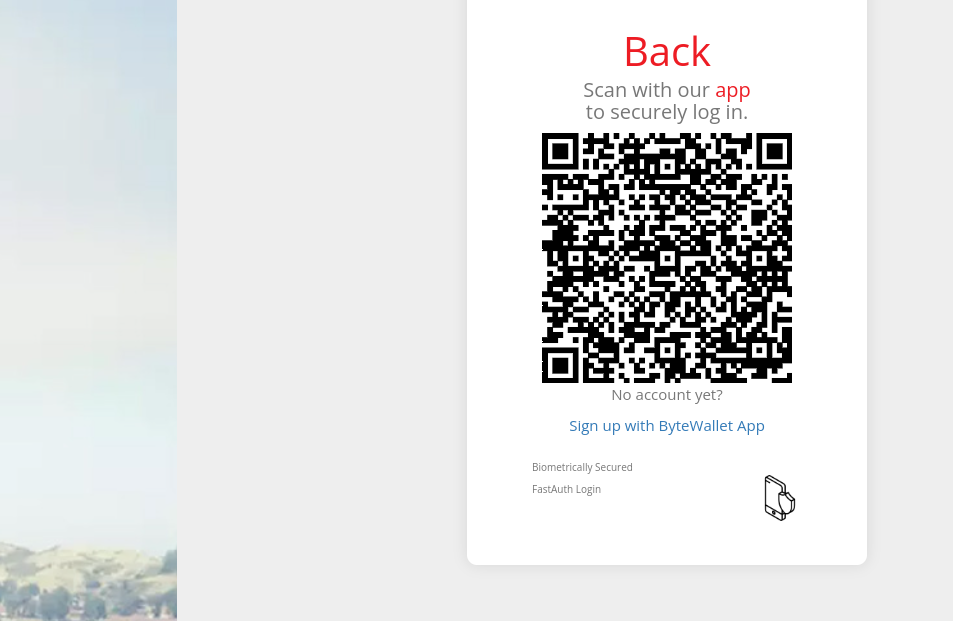
To achieve the above, here some sample CSS code we used to introduce Byteauth to a basic Laravel login screen:
.note {
position: relative;
width: 400px;
padding: 45px;
text-align: justify;
overflow: hidden;
border-radius: 10px;
}
.note:before {
content: "";
display: block;
width: 0;
position: absolute;
top: -1px;
right: -1px;
border: 30px solid transparent;
border-bottom-left-radius: 10px;
border-left: 30px solid #fff;
border-bottom: 30px solid #fff;
box-shadow: 0px 2px 4px rgba(0,0,0,0.4), -1px 1px 4px rgba(0,0,0,0.4);
background-color: #eee;
}
.note .qr-icon {
position: absolute;
top: 5px;
right: 5px;
width: 22px;
height: 22px;
background: url('/assets/img/qricon.png') no-repeat center center;
background-size: cover;
}
.login-container .loginbox {
position: relative;
height: auto !important;
padding: 0 0 20px 0;
}
.cardBack {
display: none;
}
.cardBack.flipped {
display: block;
width: 400px;
padding: 45px;
border-radius: 10px;
}
.cardFront.flipped {
display: none;
}
.shield {
margin: 0;
padding: 3px 0 3px 23px;
list-style: none;
display: flex;
align-items: center;
}
.spinner {
-webkit-animation: rotator 1.4s linear infinite;
animation: rotator 1.4s linear infinite;
}
and html:
<form method="POST" action="{{ route('login') }}">
@csrf
<div id="cardFront" class="loginbox bg-white note cardFront">
<a href="#" id="flip-btn" class="qr-icon" ></a>
<div class="registerbox-title" style="text-align: center;margin-bottom: 35px;">
<a target="_blank" href="/"><img src="/assets/img/logo-rs.png" alt="" style="height: 50px;margin-top: 25px;" /></a>
</div>
<div class="loginbox-textbox">
<input id="email" class="form-control" placeholder="Enter Email" type="email" name="email" :value="old('email')" required autofocus autocomplete="username" />
<x-input-error :messages="$errors->get('email')" class="mt-2" />
</div>
<div class="loginbox-textbox">
<input type="password" class="form-control" placeholder="Enter Password" id="password" name="password" required autocomplete="new-password" />
<x-input-error :messages="$errors->get('password')" class="mt-2" />
</div>
<div class="loginbox-forgot">
@if (Route::has('password.request'))
<a href="{{ route('password.request') }}">Forgot Password?</a>
@endif
</div>
<div class="loginbox-submit">
<input style="font-size: 18px;" type="submit" class="btn btn-primary btn-block btn-lg" value="Login">
</div>
<div class="loginbox-signup">
<a href="/register">New here? Join us!</a>
</div>
</div>
<div id="cardBack" class="loginbox bg-white cardBack" >
<div class="card-body">
<div class="auth-full-page-content rounded d-flex p-3 my-2" bis_skin_checked="1">
<div class="w-100" bis_skin_checked="1">
<div class="d-flex flex-column h-100" bis_skin_checked="1">
<div class="auth-content my-auto" bis_skin_checked="1">
<div class="text-center" bis_skin_checked="1">
<a href="#" id="flip-btn4" class="mb-0 fw-semibold" style="font-size: 40px; color: #ed1c24;">Back</a>
<p style="font-size: 20px;" class="text-muted">
Scan with our <a class="fw-semibold" id="flip-btn" style="color: #ed1c24;" href="https://bytefed.us/wallet">app</a><br> to securely log in.
</p>
</div>
<div class="mt-4 pt-3 text-center" bis_skin_checked="1">
<div class="d-flex justify-content-center mt-3">
<div class="p-2 border rounded">
@livewire('q-r-login')
</div>
</div>
</div>
<div class="mt-4 pt-3 text-center" bis_skin_checked="1">
<p style="font-size: 15px;" class="text-muted mb-1">
No account yet?
</p>
<a class="link-primary" href="https://bytefed.us/wallet" style="cursor: pointer; font-size: 15px;">
Sign up with ByteWallet App
</a>
</div>
</div>
</div>
</div>
</div>
</div>
<div class="text-left" bis_skin_checked="1">
<img src="https://img.freepik.com/free-icon/smartphone_318-548779.jpg" style="float: right;height: 46px;margin-right: 6px;/* margin-bottom: 10px; */margin: 19px;">
<p style="font-size: 10px;margin: 20px;" class="text-muted ">Biometrically Secured<br> FastAuth
Login</p>
</div>
</div>
</form>